效果如下:
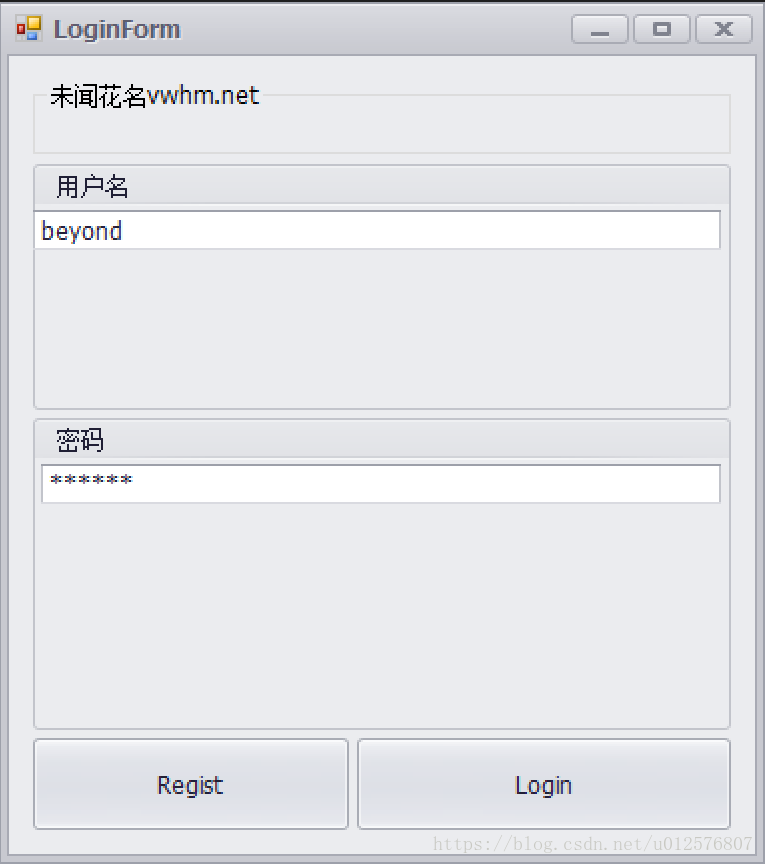
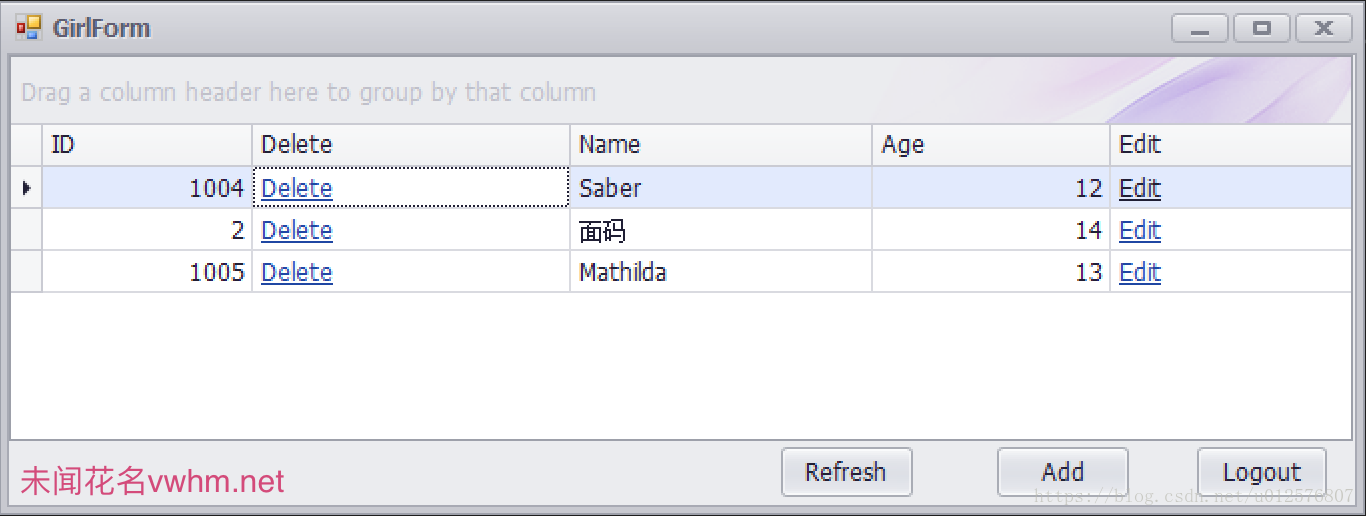
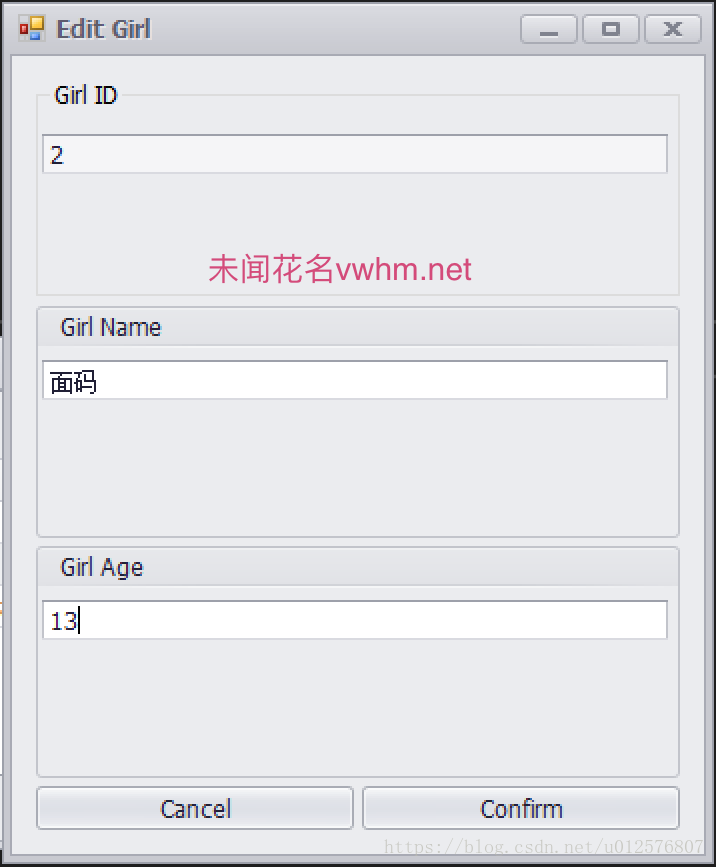
实现功能如下:
1. 登录验证(含自动登录)
2. 列表展示
3. 删除记录
4. 新增记录
5. 修改记录
用到的控件和技术如下:
1. 登录界面布局(使用LayoutControl + 容器GroupBox + TextEdit + SimpleButton)
2. 启动时判断是否之前登录过,如果登录过,直接进入列表界面
3. 登录成功后跳转(并保存登录状态到缓存中)
4. GridControl中的GridView列表数据源绑定(使用数据源配置向导绑定db5库中的Girl表)
5. GridControl控件上右击,弹出菜单中选择Run Designer,
来到设计界面,设置属性Repository (In-place EditorRepository),
添加两个行内的操作:删除和修改,如下图所示:
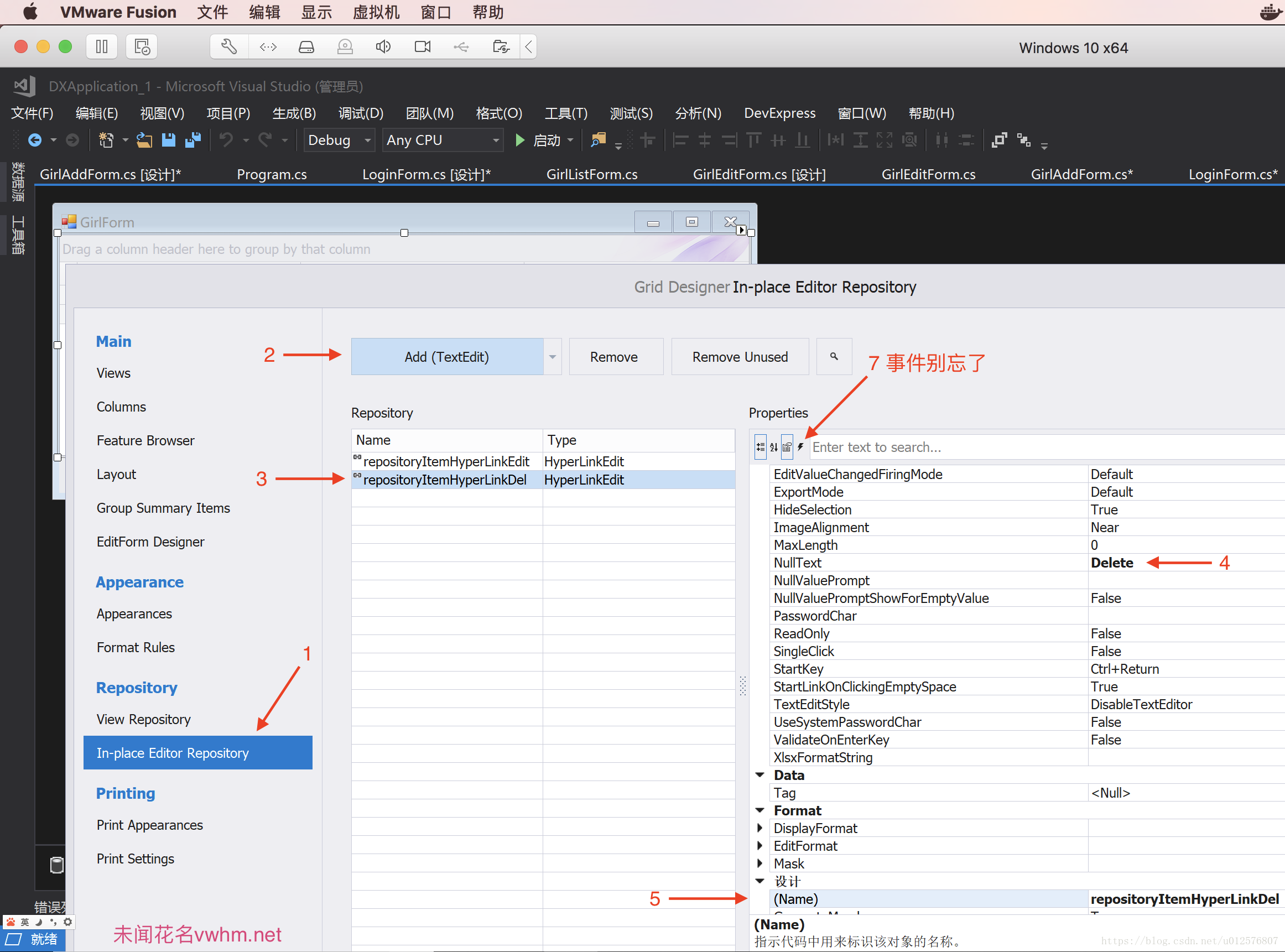
6. 实现行内删除点击事件(闪电)
7. 在删除的点击事件中,获取当前用户点击的行号
8. 连接数据库执行删除操作,并更新GridView界面
9. 点击界面上的新增按钮,弹出新增界面,
并等待新增界面返回的DialogResult,如果是OK,则刷新列表
10. 使用LayoutControl布局 新增界面,
监听点击事件,判断非空条件,连接数据库,完成添加操作
设置返回值DialogResult为OK,最后Close新增界面
11. 点击行内的修改按钮,获取用户点击的行的记录的ID
赋值修改界面,并启动修改界面
12. 修改界面根据ID查询数据库,回显数据到界面
13. 修改界面点击保存按钮, 进行非空检测,并写入数据库,
最后设置DialogResult为OK,关闭修改界面
项目结构如下:
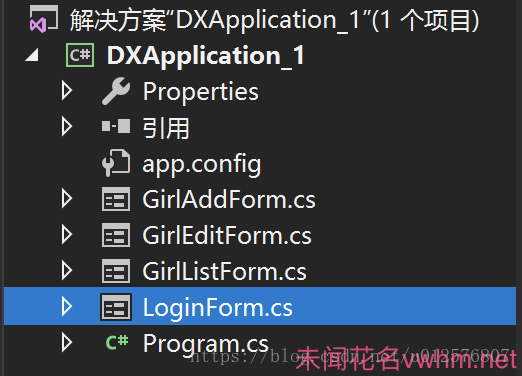
Github下载代码:
https://github.com/ixixii/DevExpress_CRUD_Demo
完整代码如下:
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using DevExpress.UserSkins;
using DevExpress.Skins;
using DevExpress.LookAndFeel;
using System.IO;
namespace DXApplication_1
{
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
BonusSkins.Register();
SkinManager.EnableFormSkins();
UserLookAndFeel.Default.SetSkinStyle("DevExpress Style");
// read login state
if (File.Exists("login.txt")) {
var savedStr = File.ReadAllText("login.txt");
if (savedStr == "HasLogin")
{
Application.Run(new GirlListForm());
return;
}
}
LoginForm loginForm = new LoginForm();
loginForm.ShowDialog();
if (loginForm.DialogResult == DialogResult.OK) {
Application.Run(new GirlListForm());
}
}
}
}
LoginForm.cs代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using DevExpress.XtraEditors;
using System.IO;
namespace DXApplication_1
{
public partial class LoginForm : DevExpress.XtraEditors.XtraForm
{
public LoginForm()
{
InitializeComponent();
}
private void simpleButton1_Click(object sender, EventArgs e)
{
var userName = textEdit1.EditValue.ToString();
var password = textEdit2.EditValue.ToString();
if (userName == "beyond" && password =="123456") {
// save login state
File.AppendAllText("login.txt", "HasLogin");
this.DialogResult = DialogResult.OK;
this.Close();
}
else {
MessageBox.Show("username or password is wrong");
}
}
private void LoginForm_Load(object sender, EventArgs e)
{
}
}
}
GirlListForm.cs代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using DevExpress.XtraEditors;
using System.IO;
using DevExpress.XtraGrid.Columns;
using System.Data.SqlClient;
namespace DXApplication_1
{
public partial class GirlListForm : DevExpress.XtraEditors.XtraForm
{
public GirlListForm()
{
InitializeComponent();
// This line of code is generated by Data Source Configuration Wizard
// Fill a SqlDataSource
sqlDataSource1.Fill();
}
private void GirlForm_Load(object sender, EventArgs e)
{
// delete column
GridColumn delColumn = new GridColumn();
delColumn.Caption = "Delete";
delColumn.Visible = true;
delColumn.ColumnEdit = repositoryItemHyperLinkDel;
gridView1.Columns.Add(delColumn);
delColumn.VisibleIndex = 4;
gridView1.BestFitColumns();
// edit column
GridColumn editColumn = new GridColumn();
editColumn.Caption = "Edit";
editColumn.Visible = true;
editColumn.ColumnEdit = repositoryItemHyperLinkEdit;
gridView1.Columns.Add(editColumn);
editColumn.VisibleIndex = 5;
gridView1.BestFitColumns();
}
private void gridControl1_Click(object sender, EventArgs e)
{
}
// logout Btn Clicked
private void simpleButton1_Click(object sender, EventArgs e)
{
File.Delete("login.txt");
LoginForm loginForm = new LoginForm();
loginForm.ShowDialog();
this.Close();
}
// delete column clicked
private void repositoryItemHyperLinkDel_Click(object sender, EventArgs e)
{
if (MessageBox.Show("Confirm Deletion?","vwhm.net",MessageBoxButtons.OKCancel)==DialogResult.OK)
{
// Get Selected Row
int[] selectedRows = gridView1.GetSelectedRows();
int selectedIndex = selectedRows[0];
var idStr = gridView1.GetRowCellValue(selectedIndex, "ID");
// write sql, Server=DESKTOP-1G3JHJP;
var connStr = "server=.;database=db5;Integrated Security=true;";
SqlConnection conn = new SqlConnection(connStr);
SqlCommand cmd = conn.CreateCommand();
cmd.CommandText = "delete from Girl where ID = @ID";
cmd.Parameters.Clear();
cmd.Parameters.Add("@ID", idStr);
conn.Open();
cmd.ExecuteNonQuery();
cmd.Dispose();
conn.Close();
// refresh data source
sqlDataSource1.Fill();
}
}
// refresh button clicked
private void simpleButton3_Click(object sender, EventArgs e)
{
// gridControl1.DataSource = list;
// gridView1.RefreshData();
sqlDataSource1.Fill();
}
// add Btn Clicked
private void simpleButton2_Click(object sender, EventArgs e)
{
// 弹出窗体,新增,保存,刷新
GirlAddForm addForm = new GirlAddForm();
addForm.ShowDialog();
if (addForm.DialogResult == DialogResult.OK) {
sqlDataSource1.Fill();
}
}
// edit column clicked
private void repositoryItemHyperLinkEdit_Click(object sender, EventArgs e)
{
// get selected Row : ID Age Name
int[] selectedRows = gridView1.GetSelectedRows();
int selectedIndex = selectedRows[0];
var idStr = gridView1.GetRowCellValue(selectedIndex, "ID");
// bind Data to Edit Form
// 弹出窗体, Modify,保存,刷新
GirlEditForm editForm = new GirlEditForm();
editForm.ID = idStr.ToString();
editForm.ShowDialog();
// Wait Edit Form DialogResult and Refresh GridView
if (editForm.DialogResult == DialogResult.OK)
{
sqlDataSource1.Fill();
}
}
}
}
GirlAddForm.cs代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using DevExpress.XtraEditors;
using System.Data.SqlClient;
namespace DXApplication_1
{
public partial class GirlAddForm : DevExpress.XtraEditors.XtraForm
{
public GirlAddForm()
{
InitializeComponent();
}
// Cancel Btn Clicked
private void simpleButton2_Click(object sender, EventArgs e)
{
this.Close();
}
// Add Btn Clicked
private void simpleButton1_Click(object sender, EventArgs e)
{
// get input
if (textEdit1_name.EditValue == null || textEdit2_age.EditValue == null)
{
MessageBox.Show("Empty Not Allowed");
return;
}
var girlName = textEdit1_name.EditValue.ToString();
var girlAge = textEdit2_age.EditValue.ToString();
if (String.IsNullOrEmpty(girlName) || String.IsNullOrEmpty(girlAge)) {
MessageBox.Show("Empty Not Allowed");
return;
}
// insert db
// write sql, Server=DESKTOP-1G3JHJP;
var connStr = "server=.;database=db5;Integrated Security=true;";
SqlConnection conn = new SqlConnection(connStr);
SqlCommand cmd = conn.CreateCommand();
cmd.CommandText = "insert into Girl values (@Name,@Age)";
cmd.Parameters.Clear();
cmd.Parameters.Add("@Name", girlName);
cmd.Parameters.Add("@Age", girlAge);
conn.Open();
cmd.ExecuteNonQuery();
cmd.Dispose();
conn.Close();
// close form and tell parent to refresh
this.DialogResult = DialogResult.OK;
this.Close();
}
private void GirlAddForm_Load(object sender, EventArgs e)
{
}
}
}
GirlEditForm.cs代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using DevExpress.XtraEditors;
using System.Data.SqlClient;
namespace DXApplication_1
{
public partial class GirlEditForm : DevExpress.XtraEditors.XtraForm
{
public string ID { get; set; }
public GirlEditForm()
{
InitializeComponent();
}
private void simpleButton1_Click(object sender, EventArgs e)
{
this.Close();
}
private void GirlEditForm_Load(object sender, EventArgs e)
{
// connect to sql
var connStr = "server=.;database=db5;Integrated Security=true;";
SqlConnection conn = new SqlConnection(connStr);
// select by idstr
SqlCommand cmd = conn.CreateCommand();
cmd.CommandText = "select ID,Name,Age from Girl where ID=@ID";
cmd.Parameters.Clear();
cmd.Parameters.Add("@ID", this.ID);
conn.Open();
//------------------
SqlDataReader reader = cmd.ExecuteReader();
if (reader.HasRows) {
while (reader.Read()) {
// bind value
textEdit1.EditValue = reader["ID"].ToString();
textEdit2.EditValue = reader["Name"].ToString();
textEdit3.EditValue = reader["Age"].ToString();
}
}
//------------------
cmd.Dispose();
conn.Close();
}
private void simpleButton2_Click(object sender, EventArgs e)
{
// connect to sql
var connStr = "server=.;database=db5;Integrated Security=true;";
SqlConnection conn = new SqlConnection(connStr);
// select by idstr
SqlCommand cmd = conn.CreateCommand();
cmd.CommandText = "update Girl set Name = @Name,Age = @Age where ID=@ID";
cmd.Parameters.Clear();
cmd.Parameters.Add("@ID", this.ID);
cmd.Parameters.Add(@"Name", textEdit2.EditValue);
cmd.Parameters.Add(@"Age", textEdit3.EditValue);
conn.Open();
//------------------
cmd.ExecuteNonQuery();
//------------------
// close form and tell parent to refresh
this.DialogResult = DialogResult.OK;
this.Close();
}
}
}